from sympy import *
import numpy as np
from tabulate import tabulate
import pandas as pd
import matplotlib.pyplot as plt
import SymMNA
from IPython.display import display, Markdown, Math, Latex
init_printing()
27 Initial conditions
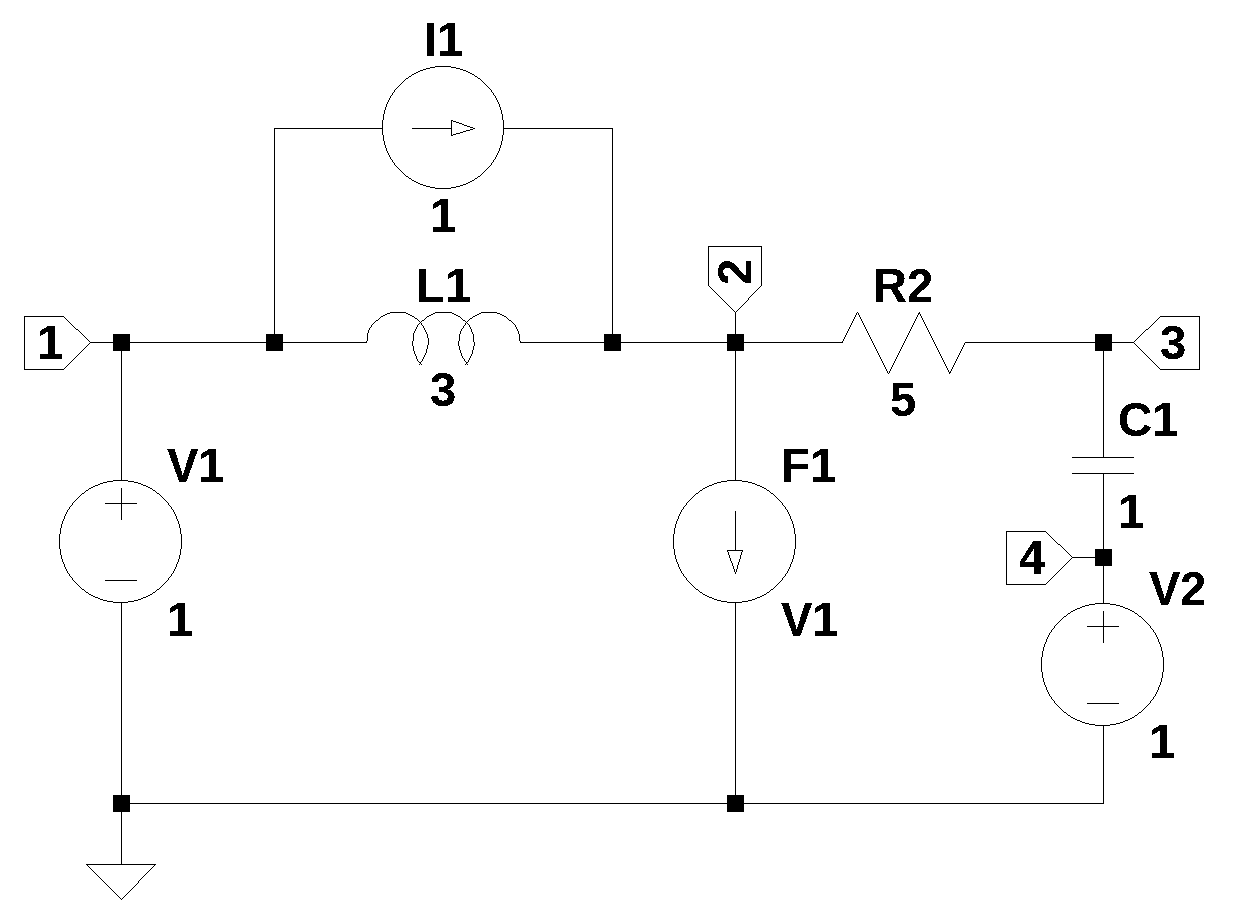
27.1 Introduction
A circuit with initial conditions consisting of a capacitor with an inital voltage and an inductor with initial current is analysed.
27.2 Circuit description
The circuit in Figure 27.1 has seven branches and four nodes. Capacitor, C1, has an initial voltage at t(0) and the inductor, L1, has an initial current at t(0). The voltage source V2 represents the initial voltage on the capacitor and current source, I1, represents the initial current flowing in the inductor. The circuit has a DC voltage source, V1. There a current controlled current source, F1, that is controlled by the current in V1.
27.3 Circuit analysis
The circuit was drawn using LTSpice and the netlist was pasted into the code. The Heaviside function is used to define the initial current and voltage on the inductor and capacitor. The Heaviside function is named after Oliver Heaviside, who made significant contributions to electrical engineering.
The following Python modules are used.
= symbols('t',positive=True) # t > 0 t
27.3.1 Load the netlist
The netlist generated by LTSpice is pasted into the cell below and some edits were made to remove the inductor series resistance and the independent sources are set to their DC values.
= '''
net_list V1 1 0 1
R2 3 2 5
C1 3 4 1
V2 4 0 1
L1 1 2 3
I1 1 2 1
F1 2 0 V1 2
'''
Generate the network equations.
= SymMNA.smna(net_list)
report, network_df, df2, A, X, Z
# Put matricies into SymPy
= Matrix(X)
X = Matrix(Z)
Z
= Eq(A*X,Z) NE_sym
Generate markdown text to display the network equations.
= ''
temp for i in range(len(X)):
+= '${:s}$<br>'.format(latex(Eq((A*X)[i:i+1][0],Z[i])))
temp
Markdown(temp)
\(I_{L1} + I_{V1} = - I_{1}\)
\(I_{F1} - I_{L1} + \frac{v_{2}}{R_{2}} - \frac{v_{3}}{R_{2}} = I_{1}\)
\(- C_{1} s v_{4} + v_{3} \left(C_{1} s + \frac{1}{R_{2}}\right) - \frac{v_{2}}{R_{2}} = 0\)
\(- C_{1} s v_{3} + C_{1} s v_{4} + I_{V2} = 0\)
\(v_{1} = V_{1}\)
\(v_{4} = V_{2}\)
\(- I_{L1} L_{1} s + v_{1} - v_{2} = 0\)
\(I_{F1} - I_{V1} f_{1} = 0\)
As shown above MNA generated many equations and these would be difficult to solve by hand and a symbolic soultion would take a lot of computing time. The equations are displace in matrix notation.
NE_sym
\(\displaystyle \left[\begin{matrix}I_{L1} + I_{V1}\\I_{F1} - I_{L1} + \frac{v_{2}}{R_{2}} - \frac{v_{3}}{R_{2}}\\- C_{1} s v_{4} + v_{3} \left(C_{1} s + \frac{1}{R_{2}}\right) - \frac{v_{2}}{R_{2}}\\- C_{1} s v_{3} + C_{1} s v_{4} + I_{V2}\\v_{1}\\v_{4}\\- I_{L1} L_{1} s + v_{1} - v_{2}\\I_{F1} - I_{V1} f_{1}\end{matrix}\right] = \left[\begin{matrix}- I_{1}\\I_{1}\\0\\0\\V_{1}\\V_{2}\\0\\0\end{matrix}\right]\)
The symbols generated by the Python code are extraced by the SymPy function free_symbols and then declared as SymPy variables.
# turn the free symbols into SymPy variables
str(NE_sym.free_symbols).replace('{','').replace('}','')) var(
\(\displaystyle \left( f_{1}, \ C_{1}, \ L_{1}, \ I_{V1}, \ I_{L1}, \ v_{2}, \ R_{2}, \ v_{4}, \ V_{2}, \ v_{1}, \ V_{1}, \ I_{F1}, \ s, \ I_{V2}, \ v_{3}, \ I_{1}\right)\)
Built a dictionary of element values.
= SymMNA.get_part_values(network_df)
element_values element_values
\(\displaystyle \left\{ C_{1} : 1.0, \ I_{1} : 1.0, \ L_{1} : 3.0, \ R_{2} : 5.0, \ V_{1} : 1.0, \ V_{2} : 1.0, \ f_{1} : 2.0\right\}\)
27.4 Initial conditions
The SymPy Heaviside function is used to define the initial current and voltage on the inductor and capacitor.
= laplace_transform(Heaviside(t), t, s)[0]
element_values[V1] = laplace_transform(-0.2*Heaviside(t), t, s)[0]
element_values[V2] = laplace_transform(0.1*Heaviside(t), t, s)[0]
element_values[I1] = NE_sym.subs(element_values)
NE_ic NE_ic
\(\displaystyle \left[\begin{matrix}I_{L1} + I_{V1}\\I_{F1} - I_{L1} + 0.2 v_{2} - 0.2 v_{3}\\- 1.0 s v_{4} - 0.2 v_{2} + v_{3} \cdot \left(1.0 s + 0.2\right)\\I_{V2} - 1.0 s v_{3} + 1.0 s v_{4}\\v_{1}\\v_{4}\\- 3.0 I_{L1} s + v_{1} - v_{2}\\I_{F1} - 2.0 I_{V1}\end{matrix}\right] = \left[\begin{matrix}- \frac{0.1}{s}\\\frac{0.1}{s}\\0\\0\\\frac{1}{s}\\- \frac{0.2}{s}\\0\\0\end{matrix}\right]\)
Solve the network equations and display the results.
= solve(NE_ic,X)
U_ic
= ''
temp for i in U_ic.keys():
+= '${:s} = {:s}$<br>'.format(latex(i),latex(U_ic[i]))
temp
Markdown(temp)
\(v_{1} = \frac{1}{s}\)
\(v_{2} = \frac{13.0 s^{2} + 53.0 s + 10.0}{10.0 s^{3} + 50.0 s^{2} + 10.0 s}\)
\(v_{3} = \frac{- 2.0 s^{2} - 7.0 s + 10.0}{10.0 s^{3} + 50.0 s^{2} + 10.0 s}\)
\(v_{4} = - \frac{0.2}{s}\)
\(I_{V1} = \frac{- s - 4.0}{10.0 s^{2} + 50.0 s + 10.0}\)
\(I_{V2} = \frac{3.0 s + 12.0}{10.0 s^{2} + 50.0 s + 10.0}\)
\(I_{L1} = \frac{- s - 1.0}{10.0 s^{3} + 50.0 s^{2} + 10.0 s}\)
\(I_{F1} = \frac{- s - 4.0}{5.0 s^{2} + 25.0 s + 5.0}\)
The voltage on node 2 is symplified with the chain of operators applied to the expression; nsimplify(), simplify(), expand() and together(). This helps SymPy solve the inverse Laplace transform.
= U_ic[v2].nsimplify().simplify().expand().together()
temp temp
\(\displaystyle \frac{13 s^{2} + 53 s + 10}{10 s \left(s^{2} + 5 s + 1\right)}\)
= inverse_laplace_transform(temp, s, t)
node_2_voltage node_2_voltage
\(\displaystyle \left(\frac{\sqrt{21} \cdot \left(3 e^{\frac{t \left(5 - \sqrt{21}\right)}{2}} + \sqrt{21} e^{\frac{t \left(5 - \sqrt{21}\right)}{2}} - 3 e^{\frac{t \left(\sqrt{21} + 5\right)}{2}} + \sqrt{21} e^{\frac{t \left(\sqrt{21} + 5\right)}{2}}\right)}{140} + e^{5 t}\right) e^{- 5 t}\)
= lambdify(t, node_2_voltage) func_node_2_voltage
The voltage on node 3 is obtained in a sumular way.
= U_ic[v3].nsimplify().simplify().expand().together()
temp temp
\(\displaystyle \frac{- 2 s^{2} - 7 s + 10}{10 s \left(s^{2} + 5 s + 1\right)}\)
= inverse_laplace_transform(temp, s, t)
node_3_voltage node_3_voltage
\(\displaystyle \left(\frac{\sqrt{21} \left(- 2 \sqrt{21} e^{\frac{t \left(5 - \sqrt{21}\right)}{2}} + 9 e^{\frac{t \left(5 - \sqrt{21}\right)}{2}} - 2 \sqrt{21} e^{\frac{t \left(\sqrt{21} + 5\right)}{2}} - 9 e^{\frac{t \left(\sqrt{21} + 5\right)}{2}}\right)}{70} + e^{5 t}\right) e^{- 5 t}\)
= lambdify(t, node_3_voltage) func_node_3_voltage
The plot below shows the node voltages versus time.
= np.linspace(0, 10, 2000, endpoint=True)
x
'Node voltages vs time')
plt.title(
='v2(t)')
plt.plot(x, func_node_2_voltage(x),label='v3(t)')
plt.plot(x, func_node_3_voltage(x),label
'v(t), volts')
plt.ylabel('time, sec')
plt.xlabel(
plt.legend()
plt.grid() plt.show()
27.5 Summary
In this notebook, newtork equations were solved which had initial conditions, which were included in the network equations with their Laplace equilivents.